The Essential API Security Checklist
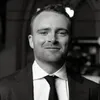
Updated April 8, 2025.

APIs are the perfect attack vector for hackers. For one, there’s no shortage of targets. There are hundreds of millions of APIs across the internet, and each has at least one endpoint that acts as a potential point of weakness. As your organization scales, you will manage the security of more internal APIs.
40% of organizations reported API security incidents in the past year—a staggering 681% jump from previous figures. APIs aren’t going anywhere, and the cybersecurity landscape isn’t getting any less risky. However, you can dramatically limit vulnerabilities and the success of standard attack mechanisms when you follow a comprehensive API security checklist.
What Is an API Security Checklist?
An API security checklist outlines essential controls you need to implement to secure your API endpoints properly. It lists measures like proper authentication, robust authorization, data encryption, input validation, rate limiting, and data minimization.
It doesn’t just provide vague advice; it creates actionable, automated rules that force accountability. You can use one to verify that every part of an API meets established best practices. A checklist can also help you pinpoint what you need from an API security tool and confirm it meets your security requirements.
Why API Security Is Mission-Critical
API ecosystems are paradoxical. They help integrate a wide range of services and scale your applications, but they significantly amplify risk. Modern applications are built on a sprawl of APIs, with API calls accounting for 71% of internet traffic. This sheer volume makes them prime targets for malicious attacks.
It also means organizations must juggle hundreds of APIs, including microservices, third-party tools, and legacy systems. All of which come with different protocols, security measures, and performance considerations. Yet each API presents itself as a possible attack vector, and it only takes one vulnerable API to poison your entire stack.
Bots worsen this situation by generating malicious API traffic, such as scraping data, guessing passwords, or exploiting authorization gaps. These attacks are carried out at scale, making it difficult for basic security measures, like account lockouts or CAPTCHA challenges, to block them effectively. Increasingly common attacks include brute force and credential stuffing, which are used to hijack legitimate user sessions.
The Essential API Security Checklist
1. Enforce Strong Authentication and Authorization
You might be using OAuth 2.0, but are you validating tokens correctly? In 2023, T-Mobile experienced a devastating API breach that lasted for weeks and exposed 37 million customer records, all because an endpoint was unprotected. There was no authorization, token validation, or checks in place.
API keys should be rotated based on their risk level and usage: high-risk keys (like payment processing) should be rotated at least monthly, while lower-risk keys can follow quarterly schedules. Implement automated key rotation and secure distribution mechanisms. Tools like Jit can automate secrets detection in your CI/CD pipeline, scanning and revoking hardcoded API keys that might have been forgotten.
Stale API keys are an easy target for attackers, so rotating them regularly (ideally every week) is essential to reduce the risk of unauthorized access.
Service-to-service APIs require mutual Transport Layer Security (mTLS). If a client cannot provide a valid certificate, block the request. Additionally, ensure tokens are only used in the intended context by validating claims like the JWT's "aud" (audience) and "iss" (issuer). For example, a token issued for api.payments.com should not work with api.users.com.
Implement proper TLS configuration:
- Enforce TLS 1.2 or 1.3 only
- Use strong cipher suites (e.g., ECDHE with AES-GCM)
- Enable HTTP Strict Transport Security (HSTS)
- Implement Certificate Transparency (CT) monitoring
- Regular certificate rotation and automated renewal
Example nginx configuration:
```nginx
ssl_protocols TLSv1.2 TLSv1.3;
ssl_ciphers ECDHE-ECDSA-AES128-GCM-SHA256:ECDHE-RSA-AES128-GCM-SHA256;
ssl_prefer_server_ciphers off;
ssl_session_tickets off;
add_header Strict-Transport-Security "max-age=63072000" always;
2. Validate and Sanitize Inputs
APIs that accept unverified data are prime targets for SQLi, XSS, and malicious payloads because attackers know how frequently corners are cut regarding input handling. If your API expects JSON, then only JSON should be allowed. You should reject requests from unexpected Content-Type headers, like XML or CSV, by returning a 415 Unsupported Media Type error. This will prevent unexpected data from being processed further.
Beyond checking for the overall format, you can specify what your API should expect. Tools like OpenAPI (formerly Swagger) let you define request validation through schemas. When a request comes through, the API gateway or validation middleware rejects it if it doesn't match these criteria. For example, you can define rules such as:
- A particular field must be strictly a 10-digit number.
- A string parameter must not be longer than 50 characters.
The data can still include malicious context even if it is in the correct format. Someone might try to inject a script by submitting <script>alert(1)</script> in a comments field. If your API stores and later displays that input without sanitizing it, it could trigger a cross-site scripting (XSS) attack. To avoid this, sanitize all input data before processing or displaying it. Libraries like OWASP’s ESAPI (for Java) can help you do this effectively.
String sanitized = ESAPI.encoder().encodeForHTML(rawInput);
3. Implement Effective Rate-Limiting
Rate limiting doesn’t just stop DDoS attacks; it is also your best defense against credential stuffing, where attackers try countless password combinations until they find one that works. Zoom’s API fell victim to this type of abuse in 2020, when attackers brute-forced up to 500,000 passwords due to an unprotected /login endpoint.
Rate limits should be tailored to your API's specific use case and endpoint behavior:
- Public endpoints: Consider 30-60 requests per minute for basic operations
- Authenticated endpoints: Scale limits based on user tiers and endpoint resource cost
- Critical endpoints (login, password reset): 5-10 requests per minute
- Exponential backoff: Implement progressive rate limiting with exponential backoff for repeated violations
Always include rate limit headers (X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Reset) in responses to help clients properly handle limits.
For your most sensitive resources like /admin, if an IP tries to access these without the proper admin cookies, it should be blocked right away.
Most web frameworks offer middleware or plugins that automatically count requests and set thresholds. For instance, if you’re working with Node.js, you can use express-rate-limit:
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // Limit each IP to 100 requests per window
});
app.use('/api/', limiter);
4. Minimize Data Collection and Retention
When designing an API or registration form, consider whether each piece of information is necessary. The more data you collect, the more data attackers can exploit. A user’s name, email, and password are all you need to create an account. Where possible, simplify and aggregate your data. For example, instead of logging every user action, you could track broader metrics like the number of clicks within an hour or day.
You should also decide on a clear data retention schedule for every type of information you handle. Once the data is no longer needed, ensure it’s securely deleted or anonymized.
5. Simplify API Error Messages
Attackers can deduce a lot from overly detailed error messages, like how your database works and what validations you’re missing. Small details, like an error mentioning “invalid token format,” can alert an attacker to potential vulnerabilities.
Return appropriately detailed error messages based on the error type:
- For client errors (400-level), provide specific validation errors to help legitimate users
- For server errors (500-level), use generic messages without internal details
- Always use correct HTTP status codes (not just 500)
- Include a correlation ID in both the response and server logs
Example:
{
"error": "Invalid request parameters",
"message": "Email format is invalid",
"correlationId": "abc-123",
"status": 400
}
Log the complete error details internally with a unique correlation ID, timestamps, and context, but ensure these logs are stored securely and have restricted access. This way, your team gets the information they need for debugging without exposing it to the outside world.
6. Automate Continuous Security Testing
Manual pen tests can take weeks. By then, your team has pushed out new endpoints and vulnerabilities. The better approach is to weave security testing directly into development. Instead of waiting for a full pentest, every pull request should trigger an automated security scan to catch issues.
You can use GitHub Actions to scan your application with Jit’s product security tools automatically. Jit’s testing provides SAST (Static Application Security Testing), DAST (Dynamic Application Security), and secrets detection coverage. For example, Jit uses ZAP to mimic real-world cyberattacks on your APIs to detect misconfigurations and security vulnerabilities.
7. Monitor API Traffic in Real-Time
In 2021, a hacker abused an API endpoint to scrape data from 700 million LinkedIn user profiles – and LinkedIn didn’t notice for months. No one was monitoring for abnormal request patterns like the same IP hammering /users endpoints 50,000 times a day.
Monitoring your API traffic is the best way to catch attackers red-handed. Effective monitoring starts with API discovery, which helps you ensure that all your APIs are accounted for and actively monitored. By discovering and cataloging your APIs, you can track each one’s activity in real time, ensuring nothing is overlooked. Deploy centralized logging solutions to consolidate traffic data and start tracking metrics like:
- Request volume spikes: Look for sudden unexpected surges in API calls for each endpoint.
- Geolocation anomalies: API requests from countries or regions where you don’t operate (or don’t have a large user base) can signal bots or attackers.
- Error rates: If you see a spike in failed requests (e.g., 401 Unauthorized, 403 Forbidden), attackers may be probing your API for vulnerabilities.
You can also set up automated rules to shut down specific attack patterns before they escalate. For instance, consider blocking IPs that hit /admin without an admin token. Consider implementing risk-based traffic rules:
- Monitor and analyze traffic patterns from cloud providers and Tor exit nodes
- Use adaptive rate limiting based on source IP reputation
- Implement CAPTCHA or additional verification for high-risk sources
- Allow legitimate API gateway and cloud service traffic based on your architecture
Strengthen Your Defenses Against Standard API Attack Methods
With incidents like T-Mobile’s breach, which exposed 37 million records, failing to secure your API is a risk you can’t afford. A comprehensive checklist will help you take action now to protect your API security before you become the next major headline.
Additionally, product security platforms like Jit help you leverage tools like OWASP ZAP to scan your API vulnerabilities after every pull request automatically. If the platform finds issues, you get code-specific remediation steps directly within GitHub.
Download the API Security Checklist now and visit Jit's homepage to discover more about how developer-centric tools can transform your API security strategy.