AI-Generated Code: The Security Blind Spot Your Team Can't Ignore
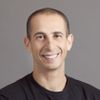
Published April 2, 2025.

The New Speed-Security Paradigm
AI coding assistants have transformed the software development landscape. Complex code that teams once deliberated for days over can now be generated code in seconds, dramatically accelerating development velocity. While this acceleration brings undeniable benefits to productivity and innovation, it creates a concerning security blind spot that organizations cannot afford to ignore.
Today's AI assistants can produce thousands of lines of code with little human oversight, often implementing complex functionality that developers themselves might not fully understand. This shift challenges our traditional security models, which assume developers have intimate knowledge of every line they deploy.
When development velocity outpaces security review capabilities, vulnerabilities will inevitably slip through.
Understanding the Security Implications of AI Coding Tools
AI coding assistants function by predicting the next most likely code sequences based on training data. This approach creates several security challenges unique to AI-generated code:
Pattern Replication
AI models replicate patterns from their training data, including insecure ones. Common vulnerabilities in open source code become templates that AI reproduces without understanding their security implications.
Incomplete Context Awareness
While AI can generate syntactically correct code, it lacks awareness of your specific application context, deployment environment, and security requirements. Without comprehensive prompting, it cannot understand how its generated code is intended to interact with your broader system architecture or security controls.
Implementation Without Understanding
Developers increasingly implement AI-suggested code they don't fully understand. This creates a growing "comprehension gap" between what's deployed and what teams actually understand, increasing the likelihood that vulnerabilities will go unnoticed.
False Sense of Authority
AI's confident presentation style creates an authority "halo effect," where developers assume code suggested by AI is inherently correct and secure, reducing critical evaluation and scrutiny.
Security-Performance Tradeoffs
AI models optimize for code that works, not necessarily code that's secure. Without explicit security prompts, they will choose the simplest implementation rather than the most secure one.
Real-World Security Vulnerabilities in AI-Generated Code
The following examples highlight common security issues that frequently appear in AI-generated code across various programming languages:
1. Java Security Flaws
// AI-generated code for user authentication
public boolean authenticateUser(String username, String password) {
String query = "SELECT * FROM users WHERE username='" + username +
"' AND password='" + password + "'";
ResultSet result = statement.executeQuery(query);
return result.next();
}
This AI-generated authentication code contains a classic SQL injection vulnerability. The AI has concatenated user input directly into the SQL query without parameterization, creating a critical security flaw that could allow attackers to bypass authentication entirely.
2. JavaScript Security Flaws
// AI-generated code for storing user preferences
function saveUserPreferences(userId, preferences) {
document.cookie = `user_${userId}_prefs=${JSON.stringify(preferences)}`;
console.log("User preferences saved!");
}
This code stores user preferences, which may include PII or sensitive configuration, in a client-side cookie without protection. It omits critical security flags like HttpOnly, Secure, and SameSite, leaving the cookie exposed to XSS, theft, and CSRF attacks. Additionally, no expiration is set, allowing indefinite access and increasing the attack surface.
3. Python Security Flaws
# AI-generated code for handling file uploads
@app.route('/upload', methods=['POST'])
def upload_file():
file = request.files['file']
filename = file.filename
file.save(os.path.join('/uploads', filename))
return 'File uploaded successfully!'
This upload handler contains multiple vulnerabilities. It blindly trusts the user-supplied filename, exposing the server to path traversal attacks (e.g., ../../etc/passwd). It performs no validation on file types or extensions, allowing potentially malicious uploads (e.g., scripts or executables). Additionally, it lacks file size limits, leaving the application vulnerable to denial-of-service attacks via oversized uploads.
4. Go Security Flaws
// AI-generated code for an API endpoint
func handleRequest(w http.ResponseWriter, r *http.Request) {
var data map[string]interface{}
decoder := json.NewDecoder(r.Body)
err := decoder.Decode(&data)
if err != nil {
fmt.Fprintf(w, "Error processing request: %v", err)
return
}
// Process data and respond
executeCommand(data["command"].(string))
w.Write([]byte("Command executed successfully"))
}
This Go example contains several vulnerabilities. It exposes raw error messages to clients, risking information disclosure. It performs no input validation and directly executes user-supplied commands, opening the door to command injection. Additionally, it uses unchecked type assertions, which can cause runtime panics if expected fields are missing or of the wrong type.
Best Practices for Secure AI-Assisted Development
The integration of AI coding assistants into development workflows requires a thoughtful approach that balances productivity with security. Organizations can significantly reduce AI-generated security risks by implementing the following best practices:
Crafting Security-Focused Prompts
How you instruct AI coding tools directly impacts the security of the generated code. Effective prompting techniques include:
Be Explicit About Security Requirements
Rather than asking for "code to handle user uploads," specify:
"secure file upload code that prevents path traversal attacks, validates file types, and limits file sizes."
AI tools generate code based on what you emphasize. If security isn’t a clear priority in prompts, it won’t be reflected in the output, leading to potential vulnerabilities.
Request Validation and Error Handling
Include phrases like "with proper input validation" and "with comprehensive error handling that doesn't leak sensitive information" in your prompts. This significantly increases the likelihood that the generated code will include these critical security elements.
Ask for Security Commentary
Prompt the AI to "include comments explaining the security considerations of this implementation" or "highlight any potential security concerns in this approach." This not only improves the generated code but also educates developers about relevant security concepts.
Use the Two-Stage Approach
First, request functional code, then follow up with "Now, identify and fix any security vulnerabilities in this code." This mimics security review processes and yields significantly more secure results than single-stage prompting.
AI-Specific Code Review Processes
Traditional code review processes must evolve to address the unique challenges of AI-generated code:
The "Comprehension Check" Review
Implement a code review process where developers explain how AI-generated code works before it can be approved. This simple step ensures that developers understand what they're implementing and catches cases where developers might blindly accept AI suggestions. It has the added benefit of teaching and training the developer.
Security-First Reviews
Implement a "security-first" pass on all AI-generated code before reviewing functionality. Since AI is generally better at coding functionality instead of security, reversing the traditional review model where functionality is reviewed first can strengthen security posture with less cost to developer cycle times.
Pattern Recognition Training
Train reviewers to recognize common AI-generated security anti-patterns. These include direct string concatenation in queries, missing input validation, and overly permissive error handling – all patterns that appear frequently in AI output.
The "Assumption Challenge" Technique For each piece of AI-generated code, explicitly list and challenge the implicit security assumptions it makes (e.g., "This code assumes user input will never contain SQL metacharacters"). AI can be used for this. This process exposes hidden vulnerabilities that traditional reviews might miss.
Comment Code with Security Requirements
AI-coding assistants often lose context rather quickly, so when working with complex and complicated codebases, having comments that outline functionality, usage context, and security requirements reinforce the security posture instructions for the AI to follow.
Developer Training for the AI Era
Equipping developers to work effectively with AI coding assistants requires new training approaches:
Security Prompt Engineering
Provide formal training on constructing security-focused prompts. Creating effective prompts is a skill that developers need to cultivate to maximize the security benefits of AI assistance.
AI Output Skepticism Training
Teach developers to maintain a healthy skepticism toward AI-generated code. Training should emphasize that AI suggestions should be treated as inputs to the development process, not authoritative outputs to be accepted without question.
Vulnerability Pattern Recognition
Train developers to recognize common vulnerability patterns in AI-generated code. This creates an additional layer of defense by making developers more likely to spot issues before code reaches review.
Regular Security Calibration Exercises
Conduct exercises where developers review intentionally vulnerable AI-generated code to identify security issues. These calibration sessions keep security awareness high and help teams stay current with evolving AI capabilities and limitations.
Tiered Approval Requirements
Implement tiered approval requirements based on the security sensitivity of the code being generated. Code interacting with authentication, payment processing, or sensitive data should require additional review layers when AI-generated.
AI-Generated Code Labeling
Require all AI-generated code to be labeled as such in comments or commit messages. This creates transparency and ensures appropriate scrutiny during review processes.
Security Verification Gates
Implementing DevSecOps best practices ensures that security is embedded throughout the development lifecycle. Establish explicit security verification gates for AI-generated code, including mandatory static analysis, dependency scanning, and in some cases, dynamic testing before deployment.
Feedback Loop Implementation
Create formal processes for feeding identified AI-generated vulnerabilities back into training and documentation. This continual improvement approach gradually reduces the occurrence of common issues.
Clear Usage Boundaries
Define explicit boundaries for when AI coding tools can and cannot be used. Some organizations prohibit AI assistance for certain security-critical components while encouraging its use for lower-risk functionality.
By implementing these best practices across prompting techniques, review processes, developer training, and organizational policies, teams can harness the productivity benefits of AI coding assistants while significantly reducing their security risks. The most successful organizations will view these practices not as overhead, but as essential components of an effective AI-augmented development strategy.
Overcoming AI-Generated Code Security Risks with Jit
The rapid adoption of AI coding assistants requires an equally sophisticated approach to security. Jit addresses the unique challenges of AI-generated code through several key capabilities:
1. Exposing Opaque Logic
AI-generated code often functions as a black box—difficult to debug or verify. Jit's static analysis tools and runtime checks expose flaws that human reviewers might miss, providing visibility into security issues across your codebase regardless of whether it was written by humans or AI.
For example, Jit's Python analysis would immediately flag the insecure file upload handler shown above, highlighting the path traversal risk and suggesting secure alternatives.
2. Balancing Speed with Safety
AI tools can produce code at a rate that far outpaces traditional manual reviews. Jit's CI/CD integration with Source Code Managers ensures every commit—including rapidly generated AI code—undergoes immediate security scanning, maintaining security without sacrificing development velocity.
This continuous scanning approach ensures that even when developers accept AI suggestions without thorough review, critical vulnerabilities don't make it to production.
3. Filling Context Gaps
AI lacks awareness of your runtime environment—it can't know that a configuration that works in development might expose vulnerabilities in production. Jit's Context Engine prioritizes risks based on actual exploitability in your specific environment, not just theoretical concerns.
This context-aware approach helps teams focus remediation efforts on the vulnerabilities that matter most in their unique deployment scenarios.
4. Managing Dependency Risks
AI coding assistants frequently recommend outdated or vulnerable libraries. Jit's software composition analysis (SCA) automatically identifies these risky dependencies, ensuring that your AI-accelerated development doesn't introduce known vulnerable components.
For instance, if an AI suggests using a cryptographic library with known weaknesses, Jit would immediately flag this during coding in the IDE or when the Pull Request is created, before the vulnerable code reaches production.
5. Maintaining Compliance
The rapid iteration enabled by AI can lead to compliance drift as teams implement features without considering regulatory requirements. Jit's full-stack coverage aligns with standards like OWASP, PCI-DSS, and SOC2, automatically identifying when AI-generated code introduces compliance violations.
Embracing AI Without Compromising Security
AI coding assistants represent a fundamental shift in how software is created and offer unprecedented productivity gains, along with introducing new security challenges. Organizations that recognize and address these challenges can harness AI's power without compromising security.
The most successful teams will implement a multi-layered strategy that includes:
Developer education on AI-specific security risks
Clear guidance on when and how to use AI coding assistants
Automated security scanning embedded in development workflows
Context-aware analysis that prioritizes relevant vulnerabilities
By integrating Jit's security automation throughout your development process, you can maintain the velocity advantages of AI-generated code while ensuring it meets your security and compliance requirements.
In a development landscape where code generation speed continues to accelerate, security automation isn't optional. It’s essential. The organizations that thrive will be those that match AI's coding capabilities with equally sophisticated security mechanisms, transforming what could be a critical blind spot into a competitive advantage.